Program to find Normal and Trace of a Square Matrix
Program to find Normal and Trace of a Square Matrix
Few important points to remember:
- Normal and Trace are only defined for a square matrix.
- Square Matrix: Matrix in which, the number of rows = number of columns.
- Normal: Square root of the sum of the squares of each element of the matrix.
- Trace: Sum of the diagonal elements of a matrix.
- Diagonal Element: An element having same indices for row and column.
Let's have a simple example to understand these terms better:
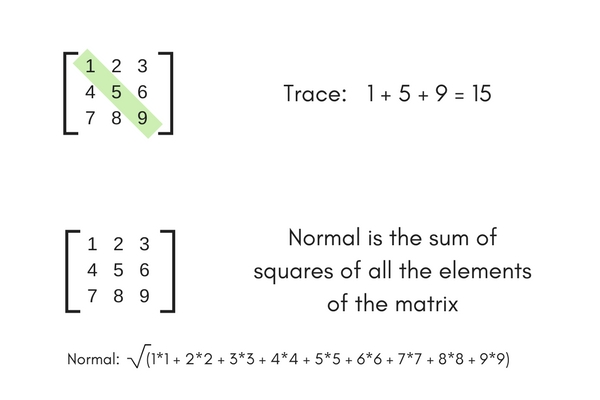
Below is a program to find Normal and Trace of Square Matrix.
#include<stdio.h>
/*
to use the sqrt method to find
the square root of a number we include
math.h header file
*/
#include<math.h>
int main()
{
printf("\n\n\t\tStudytonight - Best place to learn\n\n\n");
int i, j, n, aj[10][10], sum = 0, sum1 = 0, a = 0, normal;
printf("\nEnter the number of rows (columns) of the matrix: \n\n");
scanf("%d", &n);
printf("\nEnter the %d elements of the first matrix: \n\n", n*n);
for(i = 0; i < n; i++) // to iterate the rows
{
for(j = 0; j < n; j++) // to iterate the columns
{
scanf("%d", &aj[i][j]);
a = aj[i][j]*aj[i][j]; // finding square of each element
sum1 += a; // same as sum1 = sum1 + a
}
}
normal = sqrt((double)sum1); // typecasting to double value
printf("\n\nThe normal of the given matrix is: %d", normal);
for(i = 0; i < n; i++)
{
sum = sum + aj[i][i]; // sum of the diagonal elements
}
printf("\n\nThe Trace of the given matrix is: %d", sum);
printf("\n\n\t\t\tCoding is Fun !\n\n\n");
return 0;
}